Hi rani,
Check this example. Now please take its reference and correct your code.
Install System.Drawing.Common using the command line.
Install-Package System.Drawing.Common -Version 5.0.2
Namespaces
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Drawing.Imaging;
using System.Drawing.Text;
using System.IO;
Controller
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
[HttpPost]
public IActionResult Index(string value)
{
byte[] bytes = null;
using (var stream = new MemoryStream())
{
Bitmap bitmap = new Bitmap(250, 100, PixelFormat.Format64bppArgb);
Graphics graphics = Graphics.FromImage(bitmap);
graphics.Clear(Color.White);
graphics.SmoothingMode = SmoothingMode.AntiAlias;
graphics.TextRenderingHint = TextRenderingHint.AntiAlias;
graphics.DrawString(value, new Font("Arial", 25, FontStyle.Regular), new SolidBrush(Color.FromArgb(255, 0, 0)), new PointF(0.4F, 2.4F));
graphics.Flush();
graphics.Dispose();
bitmap.Save(stream, ImageFormat.Jpeg);
bitmap.Dispose();
bytes = stream.ToArray();
}
ViewBag.Image = "data:image/png;base64," + Convert.ToBase64String(bytes, 0, bytes.Length);
return View();
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form asp-action="Index" asp-controller="Home" method="post">
<input type="text" name="value" />
<input type="submit" value="Convert" />
</form>
@if (ViewBag.Image != null)
{
<hr />
<img alt="" src="@ViewBag.Image" />
}
</body>
</html>
Screenshot
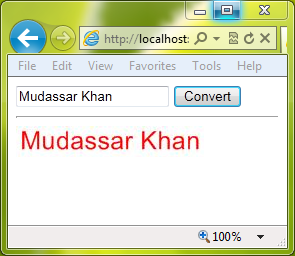