Hi Mohal,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
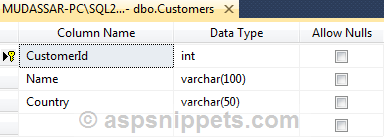
I have already inserted few records in the table.
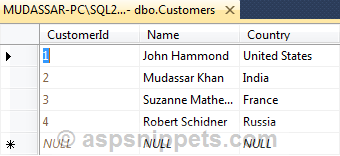
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Code
C#
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
BindGrid();
}
private void btnView_Click(object sender, EventArgs e)
{
int index = Convert.ToInt32(txtNumber.Text) - 1;
DataGridViewRow row = gvCustomers.Rows[index];
lblId.Text = row.Cells[0].Value.ToString();
lblName.Text = row.Cells[1].Value.ToString();
lblCountry.Text = row.Cells[2].Value.ToString();
}
private void BindGrid()
{
string cs = @"Server=192.168.0.100\SQL2019;Database=Test;UID=sa;PWD=pass@123";
using (SqlConnection con = new SqlConnection(cs))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM Customers", con))
{
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
gvCustomers.DataSource = dt;
}
}
}
}
}
VB.Net
Public Sub New()
InitializeComponent()
End Sub
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
BindGrid()
End Sub
Private Sub btnView_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim index As Integer = Convert.ToInt32(txtNumber.Text) - 1
Dim row As DataGridViewRow = gvCustomers.Rows(index)
lblId.Text = row.Cells(0).Value.ToString()
lblName.Text = row.Cells(1).Value.ToString()
lblCountry.Text = row.Cells(2).Value.ToString()
End Sub
Private Sub BindGrid()
Dim cs As String = "Server=192.168.0.100\SQL2019;Database=Test;UID=sa;PWD=pass@123"
Using con As SqlConnection = New SqlConnection(cs)
Using cmd As SqlCommand = New SqlCommand("SELECT * FROM Customers", con)
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
gvCustomers.DataSource = dt
End Using
End Using
End Using
End Using
End Sub
Screenshot
