Hi kankon,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
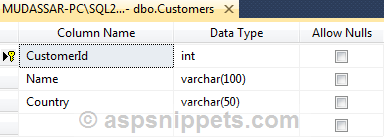
I have already inserted few records in the table.
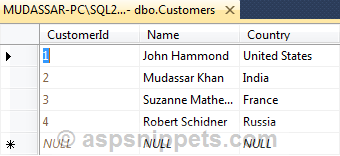
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField HeaderText="CustomerId" DataField="CustomerId" />
<asp:BoundField HeaderText="Name" DataField="Name" />
<asp:TemplateField HeaderText="Country">
<ItemTemplate>
<asp:DropDownList ID="ddlCountries" runat="server"
OnSelectedIndexChanged="OnSelectedIndexChanged"
SelectedValue='<%# Eval("Country") %>' AutoPostBack="true">
<asp:ListItem Text="India"></asp:ListItem>
<asp:ListItem Text="United States"></asp:ListItem>
<asp:ListItem Text="France"></asp:ListItem>
<asp:ListItem Text="Russia"></asp:ListItem>
</asp:DropDownList>
<br />
<asp:Button ID="Button100" runat="server" Text=" رسميى+تقرير" Visible="False"></asp:Button>
<asp:Button ID="Button200" runat="server" Text="رسميى" Visible="False"></asp:Button>
<asp:Button ID="Button300" runat="server" Text="تقرير" Visible="False"></asp:Button>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
Namespaces
C#
using System.Configuration;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindGridView();
}
}
protected void OnSelectedIndexChanged(object sender, EventArgs e)
{
DropDownList ddlCountries = sender as DropDownList;
GridViewRow row = ddlCountries.NamingContainer as GridViewRow;
Button button100 = row.FindControl("Button100") as Button;
Button button200 = row.FindControl("Button200") as Button;
Button button300 = row.FindControl("Button300") as Button;
if (ddlCountries.SelectedIndex == 1)
{
button100.Visible = true;
}
else if (ddlCountries.SelectedIndex == 2)
{
button200.Visible = true;
}
else if (ddlCountries.SelectedIndex == 3)
{
button300.Visible = true;
}
}
private void BindGridView()
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con))
{
con.Open();
gvCustomers.DataSource = cmd.ExecuteReader();
gvCustomers.DataBind();
con.Close();
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGridView()
End If
End Sub
Protected Sub OnSelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
Dim ddlCountries As DropDownList = TryCast(sender, DropDownList)
Dim row As GridViewRow = TryCast(ddlCountries.NamingContainer, GridViewRow)
Dim button100 As Button = TryCast(row.FindControl("Button100"), Button)
Dim button200 As Button = TryCast(row.FindControl("Button200"), Button)
Dim button300 As Button = TryCast(row.FindControl("Button300"), Button)
If ddlCountries.SelectedIndex = 1 Then
button100.Visible = True
ElseIf ddlCountries.SelectedIndex = 2 Then
button200.Visible = True
ElseIf ddlCountries.SelectedIndex = 3 Then
button300.Visible = True
End If
End Sub
Private Sub BindGridView()
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con)
con.Open()
gvCustomers.DataSource = cmd.ExecuteReader()
gvCustomers.DataBind()
con.Close()
End Using
End Using
End Sub
Screenshot
