Hi George616,
You need to use GridView OnRowDataBound event.
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table with the schema as follows.
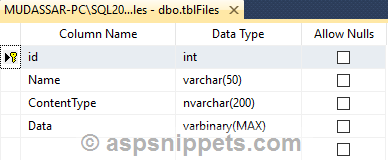
You can download the database table SQL by clicking the download link below.
Download SQL file
For more details you can refer below article.
HTML
<asp:GridView ID="GridView1" runat="server" OnRowDataBound="OnRowDataBound"
OnRowDeleting="OnRowDeleting" class="table table-bordered table-condensed table-hover" Width="100%">
<EmptyDataTemplate>
<div style="text-align: center; font-weight: bolder; font-size: medium;">
<asp:Label ID="labelTemp" runat="server" Text="No Activity Recorded"></asp:Label>
</div>
</EmptyDataTemplate>
<Columns>
<asp:TemplateField HeaderText="Image">
<ItemTemplate>
<asp:Image ID="Image1" runat="server" Width="30px" Height="30px" />
</ItemTemplate>
</asp:TemplateField>
<asp:CommandField ButtonType="Button" ShowDeleteButton="true" />
</Columns>
</asp:GridView>
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection conn = new SqlConnection(constr))
{
using (SqlDataAdapter sda = new SqlDataAdapter("SELECT Name,ContentType,Data FROM tblFiles", conn))
{
DataTable dt = new DataTable();
sda.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
}
}
protected void OnRowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
DataRowView dr = (DataRowView)e.Row.DataItem;
if (dr["ContentType"].ToString() != "image/jpeg")
{
(e.Row.Cells[1].Controls[0] as Button).Visible = false;
}
if (dr.Row.Table.Columns.Contains("Data"))
{
if (dr["Data"] != DBNull.Value)
{
string imageUrl = "data:image/jpg;base64," + Convert.ToBase64String((byte[])dr["Data"]);
(e.Row.FindControl("Image1") as Image).ImageUrl = imageUrl;
}
}
}
GridViewRow row = e.Row;
List<TableCell> cells = new List<TableCell>();
foreach (DataControlField column in GridView1.Columns)
{
TableCell cell = row.Cells[0];
row.Cells.Remove(cell);
cells.Add(cell);
}
row.Cells.AddRange(cells.ToArray());
}
protected void OnRowDeleting(object sender, GridViewDeleteEventArgs e)
{
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using conn As SqlConnection = New SqlConnection(constr)
Using sda As SqlDataAdapter = New SqlDataAdapter("SELECT Name,ContentType,Data FROM tblFiles", conn)
Dim dt As DataTable = New DataTable()
sda.Fill(dt)
GridView1.DataSource = dt
GridView1.DataBind()
End Using
End Using
End If
End Sub
Protected Sub OnRowDataBound(ByVal sender As Object, ByVal e As GridViewRowEventArgs)
If e.Row.RowType = DataControlRowType.DataRow Then
Dim dr As DataRowView = CType(e.Row.DataItem, DataRowView)
If dr("ContentType").ToString() <> "image/jpeg" Then
TryCast(e.Row.Cells(1).Controls(0), Button).Visible = False
End If
If dr.Row.Table.Columns.Contains("Data") Then
If Not IsDBNull(dr("Data")) Then
Dim imageUrl As String = "data:image/jpg;base64," & Convert.ToBase64String(CType(dr("Data"), Byte()))
TryCast(e.Row.FindControl("Image1"), Image).ImageUrl = imageUrl
End If
End If
End If
Dim row As GridViewRow = e.Row
Dim cells As List(Of TableCell) = New List(Of TableCell)()
For Each column As DataControlField In GridView1.Columns
Dim cell As TableCell = row.Cells(0)
row.Cells.Remove(cell)
cells.Add(cell)
Next
row.Cells.AddRange(cells.ToArray())
End Sub
Protected Sub OnRowDeleting(ByVal sender As Object, ByVal e As GridViewDeleteEventArgs)
End Sub
Screenshot
