Hi Rancho079,
Check this example. Now please take its reference and correct your code.
Database
For this example i have used tables Countries, State with the following schema.
Country
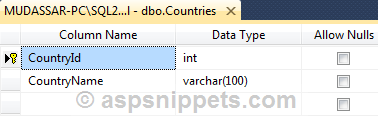
State
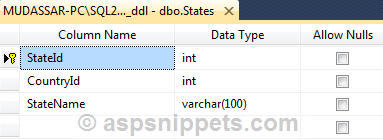
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CustomerModel
{
public int Id { get; set; }
public string Name { get; set; }
public string Country { get; set; }
public string State { get; set; }
}
Controller
public class HomeController : Controller
{
CascadingEntities entities = new CascadingEntities();
// GET: /Home/
public ActionResult Index()
{
List<CustomerModel> customers = new List<CustomerModel>();
customers.Add(new CustomerModel
{
Id = 1,
Name = "John Hammond",
State = "Alabama",
Country = "USA",
});
customers.Add(new CustomerModel
{
Id = 2,
Name = "Mudassar Khan",
State = "Maharashtra",
Country = "India",
});
return View(customers);
}
public JsonResult GetCountryList()
{
var countries = entities.Countries.Select(x => new
{
CountryId = x.CountryId,
CountryName = x.CountryName
});
return Json(countries, JsonRequestBehavior.AllowGet);
}
public JsonResult GetStateList(int country)
{
var states = entities.States.Where(x => x.CountryId == country).Select(x => new
{
StateId = x.StateId,
StateName = x.StateName
});
return Json(states, JsonRequestBehavior.AllowGet);
}
}
View
<html>
<head>
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript">
$(function () {
PopulateCountries();
$('#Country').change(function () {
// Bind States.
PopulateStates($('#Country').val());
});
// Edit Click.
$("body").on("click", "#tblCustomers .Edit", function () {
var tds = $(this).closest("tr").find('td');
$('#txtId').val($(tds).eq(0).find('span').html().trim());
$('#txtName').val($(tds).eq(1).find('span').html().trim());
var country = $(tds).eq(3).find('span').html().trim();
// Set Country option selected.
$('#Country option').each(function () {
if ($(this).html() == country) {
$(this).attr('selected', 'selected');
}
});
// Bind States.
PopulateStates($('#Country').val());
var state = $(tds).eq(2).find('span').html().trim();
// Set State option selected.
$('#State option').each(function () {
if ($(this).html() == state) {
$(this).attr('selected', 'selected');
}
});
});
});
function PopulateStates(country) {
$.ajax({
type: "GET",
url: "Home/GetStateList",
datatype: "Json",
data: { country: country },
async: false,
success: function (data) {
$('#State').html('<option value="0">Select State</option>');
var optionState = '';
for (var i = 0; i < data.length; i++) {
optionState += '<option value="' + data[i].StateId + '">' + data[i].StateName + '</option>';
}
$('#State').append(optionState);
}
});
}
function PopulateCountries() {
$.ajax({
type: "GET",
url: "Home/GetCountryList",
datatype: "Json",
data: {},
async: false,
success: function (data) {
$('#Country').html('<option value="0">Select Country</option>');
var optionCountry = '';
for (var i = 0; i < data.length; i++) {
optionCountry += '<option value="' + data[i].CountryId + '">' + data[i].CountryName + '</option>';
}
$('#Country').append(optionCountry);
}
});
}
</script>
</head>
<body>
<table id="tblCustomers" class="table table-responsive">
<tr>
<th>Id</th>
<th>Name</th>
<th>State</th>
<th>Country</th>
<th></th>
</tr>
<%foreach (CustomerModel customer in Model) {%>
<tr>
<td class="CustomerId"><span><%=customer.Id%></span></td>
<td class="Name"><span><%=customer.Name%></span></td>
<td class="State"><span><%=customer.State%></span></td>
<td class="Country"><span><%=customer.Country%></span></td>
<td><a class="Edit" href="javascript:;">Edit</a></td>
</tr>
<% } %>
</table>
<table class="table table-responsive">
<tr>
<td>Id :</td>
<td><input type="text" name="id" id="txtId" readonly="readonly" class="form-control" /></td>
</tr>
<tr>
<td>Name:</td>
<td><input type="text" name="name" id="txtName" class="form-control" /></td>
</tr>
<tr>
<td>County :</td>
<td><%:Html.DropDownList("Country", new List<SelectListItem> { }, "Select Country", new { @class = "form-control" })%></td>
</tr>
<tr>
<td>State :</td>
<td><%:Html.DropDownList("State", new List<SelectListItem> { }, "Select State", new { @class = "form-control" })%></td>
</tr>
</table>
</body>
</html>
Screenshot
