Hi kankon
You have taken the ImageButton, so you need to typecast the sender as ImageButton.
Please refer below sample.
Database
This Sample makes use of a table named tblFiles whose schema is defined as follows.
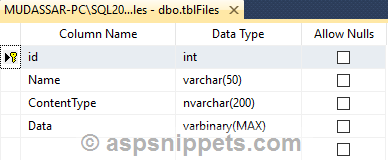
Note: You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<form id="form1" runat="server">
<div>
<link rel="stylesheet" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<asp:DataList ID="dlCustomers" runat="server" RepeatColumns="6" CellSpacing="0" RepeatLayout="Table">
<ItemTemplate>
<table class="table">
<tr>
<th colspan="2">
<b>
<%# Eval("Name") %></b>
</th>
</tr>
<tr>
<td colspan="2">
<asp:Label ID="lblId" Text='<%# Eval("id") %>' runat="server" />,
<%# Eval("ContentType") %>
</td>
</tr>
<tr>
<td>Image:
</td>
<td>
<asp:ImageButton ID="imgImage" runat="server" ImageUrl='<%# "data:image/jpg;base64," + Convert.ToBase64String((byte[])Eval("Data")) %>'
Height="75px" Width="75px" OnClick="imgImage_Click" />
</td>
</tr>
<tr>
<td colspan="2">Name:
<%# Eval("Name")%>
</td>
</tr>
<tr>
<td colspan="2">ContentType:
<%# Eval("ContentType")%>
</td>
</tr>
</table>
</ItemTemplate>
</asp:DataList>
</div>
</form>
Namespaces
C#
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
VB.Net
Imports System.Data
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
CS
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
DataTable dt = this.GetData();
dlCustomers.DataSource = dt;
dlCustomers.DataBind();
}
}
protected void imgImage_Click(object sender, EventArgs e)
{
string url = "Default.aspx?Id=" + ((sender as ImageButton).NamingContainer.FindControl("lblId") as Label).Text;
string script = "window.open('" + url + "', 'popup_window', 'width=300,height=100,left=100,top=100,resizable=yes');";
ClientScript.RegisterStartupScript(this.GetType(), "script", script, true);
}
private DataTable GetData()
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT Id,Name,ContentType,Data FROM tblFiles WHERE ContentType = 'image/jpeg'"))
{
using (SqlDataAdapter sda = new SqlDataAdapter())
{
cmd.Connection = con;
sda.SelectCommand = cmd;
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
return dt;
}
}
}
}
}
Default
protected void Page_Load(object sender, EventArgs e)
{
if (!string.IsNullOrEmpty(Request.QueryString["Id"]))
{
Response.Write("Id is : <b>" + Request.QueryString["Id"] + "</b>");
}
}
VB.Net
VB
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim dt As DataTable = Me.GetData()
dlCustomers.DataSource = dt
dlCustomers.DataBind()
End If
End Sub
Protected Sub imgImage_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim url As String = "Default.aspx?Id=" & (TryCast((TryCast(sender, ImageButton)).NamingContainer.FindControl("lblId"), Label)).Text
Dim script As String = "window.open('" & url & "', 'popup_window', 'width=300,height=100,left=100,top=100,resizable=yes');"
ClientScript.RegisterStartupScript(Me.[GetType](), "script", script, True)
End Sub
Private Function GetData() As DataTable
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT Id,Name,ContentType,Data FROM tblFiles WHERE ContentType = 'image/jpeg'")
Using sda As SqlDataAdapter = New SqlDataAdapter()
cmd.Connection = con
sda.SelectCommand = cmd
Using dt As DataTable = New DataTable()
sda.Fill(dt)
Return dt
End Using
End Using
End Using
End Using
End Function
Default
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not String.IsNullOrEmpty(Request.QueryString("Id")) Then
Response.Write("Id is : <b>" & Request.QueryString("Id") & "</b>")
End If
End Sub
Screenshot
