Please refer this example.
Database
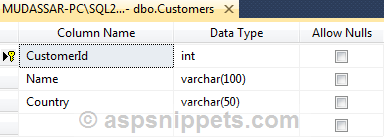
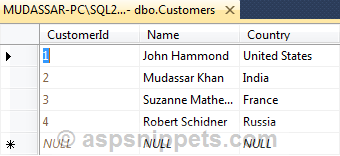
Download SQL file
using Microsoft.AspNetCore.Mvc.Rendering;
Model
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
}
Database Context
using Microsoft.EntityFrameworkCore;
using Razor_DropDownList_EF.Models;
namespace Razor_DropDownList_EF
{
public class DBCtx : DbContext
{
public DBCtx(DbContextOptions<DBCtx> options) : base(options)
{
}
public DbSet<Customer> Customers { get; set; }
}
}
Razor PageModel (Code-Behind)
namespace Razor_DropDownList_EF.Pages
{
public class IndexModel : PageModel
{
private DBCtx Context { get; }
public IndexModel(DBCtx _context)
{
this.Context = _context;
}
public SelectList Customers { get; set; }
public void OnGet()
{
this.PopulateDropDownList();
}
public void PopulateDropDownList()
{
this.Customers = new SelectList(this.Context.Customers, "CustomerId", "Name");
}
public void OnPostSubmit(string customerId)
{
this.PopulateDropDownList();
ViewData["SelectedValue"] = customerId;
}
}
}
Razor Page (HTML)
@page
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@model Razor_DropDownList_EF.Pages.IndexModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<style type="text/css">
body { font-family: Arial; font-size: 10pt; }
</style>
</head>
<body>
<form method="post">
<select id="ddlCustomers" name="CustomerId" asp-items="@Model.Customers">
<option value="0">--Select Customer--</option>
</select>
<br />
<br />
<input type="submit" value="Submit" asp-page-handler="Submit" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
@if (ViewData["SelectedValue"] != null)
{
<script type="text/javascript">
$(function () {
$(this).find("#ddlCustomers").val('@ViewData["SelectedValue"]')
});
</script>
}
</form>
</body>
</html>
Screenshot
