Hi jshorthose,
I have created sample using the article Encrypt and Decrypt Username or Password stored in database in Windows Application using C# and VB.Net.
Please refer the below example.
Database
I have made use of the following table Customers with the schema as follows.
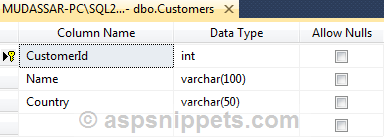
I have already inserted few records in the table.
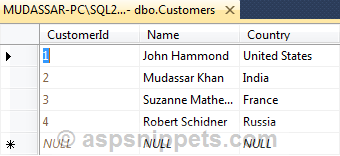
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
C#
using System.IO;
using System.Text;
using System.Data;
using System.Data.SqlClient;
using System.Security.Cryptography;
VB.Net
Imports System.IO
Imports System.Text
Imports System.Data
Imports System.Data.SqlClient
Imports System.Security.Cryptography
Code
C#
private void Form1_Load(object sender, EventArgs e)
{
string constr = @"Data Source=.\SQL2022;Initial Catalog=AjaxSamples;UID=sa;PWD=pass@123;";
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM Customers", con))
{
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
DataTable dt = new DataTable();
sda.Fill(dt);
DataTable dtCopy = new DataTable();
// Adding Columns to DataTable.
for (int i = 0; i < dt.Columns.Count; i++)
{
dtCopy.Columns.Add(dt.Columns[i].ColumnName, typeof(string));
}
// Encrypt the cell values.
foreach (DataRow row in dt.Rows)
{
DataRow dr = dtCopy.NewRow();
foreach (DataColumn column in dtCopy.Columns)
{
dr[column.ColumnName] = this.Encrypt(row[column.ColumnName].ToString());
}
dtCopy.Rows.Add(dr.ItemArray);
}
// Decrypt the cell values.
foreach (DataRow row in dtCopy.Rows)
{
foreach (DataColumn column in dtCopy.Columns)
{
row[column.ColumnName] = this.Decrypt(row[column.ColumnName].ToString());
}
}
dgvCustomers.DataSource = dtCopy;
}
}
}
}
private string Encrypt(string clearText)
{
string EncryptionKey = "MAKV2SPBNI99212";
byte[] clearBytes = Encoding.Unicode.GetBytes(clearText);
using (Aes encryptor = Aes.Create())
{
Rfc2898DeriveBytes pdb = new Rfc2898DeriveBytes(EncryptionKey, new byte[] { 0x49, 0x76, 0x61, 0x6e, 0x20, 0x4d, 0x65, 0x64, 0x76, 0x65, 0x64, 0x65, 0x76 });
encryptor.Key = pdb.GetBytes(32);
encryptor.IV = pdb.GetBytes(16);
using (MemoryStream ms = new MemoryStream())
{
using (CryptoStream cs = new CryptoStream(ms, encryptor.CreateEncryptor(), CryptoStreamMode.Write))
{
cs.Write(clearBytes, 0, clearBytes.Length);
cs.Close();
}
clearText = Convert.ToBase64String(ms.ToArray());
}
}
return clearText;
}
private string Decrypt(string cipherText)
{
string EncryptionKey = "MAKV2SPBNI99212";
byte[] cipherBytes = Convert.FromBase64String(cipherText);
using (Aes encryptor = Aes.Create())
{
Rfc2898DeriveBytes pdb = new Rfc2898DeriveBytes(EncryptionKey, new byte[] { 0x49, 0x76, 0x61, 0x6e, 0x20, 0x4d, 0x65, 0x64, 0x76, 0x65, 0x64, 0x65, 0x76 });
encryptor.Key = pdb.GetBytes(32);
encryptor.IV = pdb.GetBytes(16);
using (MemoryStream ms = new MemoryStream())
{
using (CryptoStream cs = new CryptoStream(ms, encryptor.CreateDecryptor(), CryptoStreamMode.Write))
{
cs.Write(cipherBytes, 0, cipherBytes.Length);
cs.Close();
}
cipherText = Encoding.Unicode.GetString(ms.ToArray());
}
}
return cipherText;
}
VB.Net
Private Sub Form1_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
Dim constr As String = "Data Source=.\SQL2022;Initial Catalog=AjaxSamples;UID=sa;PWD=pass@123;"
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand("SELECT * FROM Customers", con)
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Dim dt As DataTable = New DataTable()
sda.Fill(dt)
Dim dtCopy As DataTable = New DataTable()
' Adding Columns to DataTable.
For i As Integer = 0 To dt.Columns.Count - 1
dtCopy.Columns.Add(dt.Columns(i).ColumnName, GetType(String))
Next
' Encrypt the cell values.
For Each row As DataRow In dt.Rows
Dim dr As DataRow = dtCopy.NewRow()
For Each column As DataColumn In dtCopy.Columns
dr(column.ColumnName) = Me.Encrypt(row(column.ColumnName).ToString())
Next
dtCopy.Rows.Add(dr.ItemArray)
Next
' Decrypt the cell values.
For Each row As DataRow In dtCopy.Rows
For Each column As DataColumn In dtCopy.Columns
row(column.ColumnName) = Me.Decrypt(row(column.ColumnName).ToString())
Next
Next
dgvCustomers.DataSource = dtCopy
End Using
End Using
End Using
End Sub
Private Function Encrypt(clearText As String) As String
Dim EncryptionKey As String = "MAKV2SPBNI99212"
Dim clearBytes As Byte() = Encoding.Unicode.GetBytes(clearText)
Using encryptor As Aes = Aes.Create()
Dim pdb As New Rfc2898DeriveBytes(EncryptionKey, New Byte() {&H49, &H76, &H61, &H6E, &H20, &H4D, _
&H65, &H64, &H76, &H65, &H64, &H65, _
&H76})
encryptor.Key = pdb.GetBytes(32)
encryptor.IV = pdb.GetBytes(16)
Using ms As New MemoryStream()
Using cs As New CryptoStream(ms, encryptor.CreateEncryptor(), CryptoStreamMode.Write)
cs.Write(clearBytes, 0, clearBytes.Length)
cs.Close()
End Using
clearText = Convert.ToBase64String(ms.ToArray())
End Using
End Using
Return clearText
End Function
Private Function Decrypt(cipherText As String) As String
Dim EncryptionKey As String = "MAKV2SPBNI99212"
Dim cipherBytes As Byte() = Convert.FromBase64String(cipherText)
Using encryptor As Aes = Aes.Create()
Dim pdb As New Rfc2898DeriveBytes(EncryptionKey, New Byte() {&H49, &H76, &H61, &H6E, &H20, &H4D,
&H65, &H64, &H76, &H65, &H64, &H65,
&H76})
encryptor.Key = pdb.GetBytes(32)
encryptor.IV = pdb.GetBytes(16)
Using ms As New MemoryStream()
Using cs As New CryptoStream(ms, encryptor.CreateDecryptor(), CryptoStreamMode.Write)
cs.Write(cipherBytes, 0, cipherBytes.Length)
cs.Close()
End Using
cipherText = Encoding.Unicode.GetString(ms.ToArray())
End Using
End Using
Return cipherText
End Function
Screenshot
