Hi shoaibshafiqa...,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
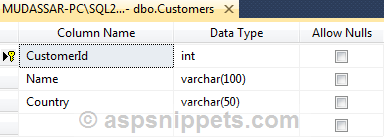
I have already inserted few records in the table.
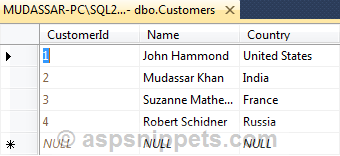
You can download the database table SQL by clicking the download link below.
Download SQL file
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
CustomerEntities entities = new CustomerEntities();
return View(entities.Customers.ToList());
}
[HttpPost]
public ActionResult Index(string search)
{
CustomerEntities entities = new CustomerEntities();
var customers = entities.Customers.ToList();
if (!string.IsNullOrEmpty(search))
{
customers = customers.Where(p => p.Name.ToLower().Contains(search.ToLower())).ToList();
}
ViewBag.Searchtext = search;
return View(customers);
}
[HttpPost]
public ActionResult Edit(int id)
{
CustomerEntities entities = new CustomerEntities();
var customer = entities.Customers.Where(x => x.CustomerId == id).FirstOrDefault();
return PartialView(customer);
}
[HttpPost]
public ActionResult EditP(Customer customer)
{
CustomerEntities entities = new CustomerEntities();
var customerUpdate = entities.Customers.Where(x => x.CustomerId == customer.CustomerId).FirstOrDefault();
customerUpdate.Name = customer.Name;
customerUpdate.Country = customer.Country;
entities.SaveChanges();
return Json("Success");
}
}
View
Index
@model List<Modal_MVC.Customer>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript" src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js"></script>
<script type="text/javascript">
$(function () {
$("[id*=BtnEdit]").click(function () {
var customerId = $(this).attr('data-id');
$.ajax({
type: 'POST',
url: '/Home/Edit',
data: { id: customerId }
}).done(function (response) {
$("#actionCon").html(response);
$("#myModalEdit").modal('show');
}).fail(function (r) {
alert(r.responsetext);
});
});
});
</script>
</head>
<body class="container">
<form method="post" action="/Home/Index">
<div class="row">
<div class="col-md-6">
<div class="form-group">
<label>Search</label>
<input class="form-control" id="searchTxt" name="Search" value="@ViewBag.Searchtext" />
</div>
</div>
<div class="col-md-4">
<div class="form-group">
<div class="row">
<div class="col-md-6">
<button class="btn btn-primary form-control" type="submit" id="searchBtn" name="search">Search</button>
</div>
</div>
</div>
</div>
</div>
</form>
<table class="table table-striped">
<thead>
<tr>
<td>Id</td>
<td>Name</td>
<td>Country</td>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>@item.CustomerId</td>
<td>@item.Name</td>
<td>@item.Country</td>
<td>
<button type="button" id="BtnEdit" data-id="@item.CustomerId">Edit</button>
</td>
</tr>
}
</tbody>
</table>
<div id="actionCon"></div>
</body>
</html>
PartialView
@model Modal_MVC.Customer
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript" src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js"></script>
<script type="text/javascript">
$(function () {
$("[id*=UpdateBtn]").click(function () {
var customer = {};
customer.CustomerId = $('[id*=hfId]').val();
customer.Name = $('[id*=txtName]').val();
customer.Country = $('[id*=txtCountry]').val();
$.ajax({
type: 'POST',
url: '/Home/EditP',
data: JSON.stringify(customer),
contentType: "application/json; charset=utf-8",
dataType: "html",
}).done(function (response) {
window.location.href = "/Home/Index";
}).fail(function (XMLHttpRequest, textStatus, errorThrown) {
});
});
});
</script>
<form id="EditCat">
<div class="container">
<!-- The Modal -->
<div class="modal fade" id="myModalEdit">
<div class="modal-dialog modal-dialog-centered">
<div class="modal-content">
<!-- Modal Header -->
<div class="modal-header">
<h4 class="modal-title">Edit Category</h4>
<button type="button" class="close" data-dismiss="modal">×</button>
</div>
<!-- Modal body -->
<div class="modal-body">
<input id="hfId" type="hidden" name="ID" value="@Model.CustomerId" />
<div class="form-group">
<input type="text" value="@Model.Name" class="form-control" id="txtName" name="Name" placeholder="Enter Name" />
</div>
<br />
<div class="form-group">
<input type="text" value="@Model.Country" class="form-control" id="txtCountry" name="Name" placeholder="Enter Country" />
</div>
</div>
<!-- Modal footer -->
<div class="modal-footer">
<button id="UpdateBtn" type="button" class="btn btn-primary" data-dismiss="modal">Update</button>
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
</div>
</form>
Screenshot
