Hi rani,
In TagHelpers you need to use select tag that is the alternative of Html.ListBoxFor.
Check this example. Now please take its reference and correct your code.
Database
For this example I have used table named Fruits whose schema is defined as follows.
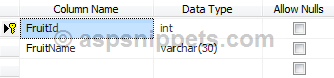
The Fruits table has the following records.
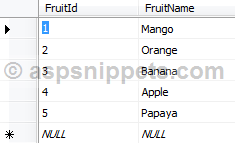
You can download the database table SQL by clicking the download link below.
Download SQL file
For entity framework configuration refer below article.
Model
public class Fruit
{
public int FruitId { get; set; }
public string FruitName { get; set; }
}
Namepsaces
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
List<SelectListItem> fruits = (from fruit in this.Context.Fruits
select new SelectListItem
{
Text = fruit.FruitName,
Value = fruit.FruitId.ToString()
}).ToList();
ViewBag.Fruits = fruits;
return View();
}
[HttpPost]
public IActionResult Index(string lstFruits)
{
var fruits = (from fruit in this.Context.Fruits
select new SelectListItem
{
Text = fruit.FruitName,
Value = fruit.FruitId.ToString()
}).ToList();
ViewBag.Fruits = fruits;
string[] fruitIds = Request.Form["lstFruits"].ToString().Split(",");
foreach (string id in fruitIds)
{
if (!string.IsNullOrEmpty(id))
{
string name = ((List<SelectListItem>)ViewBag.Fruits)
.Where(x => x.Value == id).FirstOrDefault().Text;
ViewBag.Message += "Id: " + id + " Fruit Name: " + name + "\\n";
}
}
return View();
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/css/bootstrap.min.css" />
</head>
<body>
<form method="post" asp-action="Index" asp-controller="Home">
<div class="container">
<div class="row">
<div class="col-md-2">
Fruits:
<select id="lstFruits" name="lstFruits" asp-items="ViewBag.Fruits" multiple
class="form-control" size="5"></select>
<br />
<input type="submit" value="Submit" class="btn btn-primary" />
</div>
</div>
</div>
</form>
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
};
</script>
}
</body>
</html>
Screenshot
