Hi jovceka,
To display loader on ajax call use beforeSend and complete events.
For more details refer below article.
Check this example. Now please take its reference and correct your code.
Database
I have made use of a table named tblFiles whose schema is defined as follows.
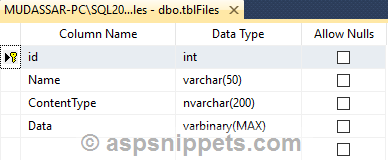
I have already inserted few records in the table.
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<style type="text/css">
.modal
{
position: fixed;
z-index: 999;
height: 100%;
width: 100%;
top: 0;
left: 0;
background-color: Black;
filter: alpha(opacity=60);
opacity: 0.6;
-moz-opacity: 0.8;
}
.center
{
z-index: 1000;
margin: 300px auto;
padding: 10px;
width: 130px;
background-color: White;
border-radius: 10px;
filter: alpha(opacity=100);
opacity: 1;
-moz-opacity: 1;
}
.center img
{
height: 128px;
width: 128px;
}
</style>
<script type="text/javascript">
$(function () {
$.ajax({
type: "POST",
contentType: "application/json; charset=utf-8",
url: "WebService.asmx/GetProducts",
dataType: "json",
success: function (data) {
for (var i = 0; i < data.d.length; i++) {
var id = data.d[i].Id;
var name = data.d[i].Name;
var image = "data:image/jpg;base64," + data.d[i].ProdImage;
$("#tblImages").append(
"<div class=trclass>" +
" <tr><td class=tdcolumn>" +
" <div class=tabledivprod>" +
" <img class=pdtimgclnt src='" + image + "' />" +
" <div class=Productname>(" + name + ")</div>" +
" <div class=PdtID>" + id + "</div>" +
" <br /><br />" +
" </div>" +
" </td></tr>" +
"</div>");
}
},
beforeSend: function () {
$(".modal").show();
},
complete: function () {
$(".modal").hide();
},
error: function (response) {
alert(response.responseText);
}
});
});
</script>
<div id="tblImages">
</div>
<div class="modal" style="display: none">
<div class="center">
<img alt="" src="Loader.gif" />
</div>
</div>
WebService
C#
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Services;
/// <summary>
/// Summary description for WebService
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class WebService : System.Web.Services.WebService
{
[WebMethod]
public List<Product> GetProducts()
{
List<Product> products = new List<Product>();
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT TOP 3 * FROM tblFiles WHERE ContentType = 'image/jpeg'";
using (SqlConnection con = new SqlConnection(conString))
{
SqlCommand cmd = new SqlCommand(query, con);
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
while (sdr.Read())
{
products.Add(new Product
{
Id = Convert.ToInt32(sdr["Id"]),
Name = sdr["Name"].ToString(),
ProdImage = Convert.ToBase64String((byte[])sdr["Data"], 0, ((byte[])sdr["Data"]).Length)
});
}
con.Close();
}
return products;
}
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public string ProdImage { get; set; }
}
}
VB.Net
Imports System
Imports System.Collections.Generic
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Web.Services
' To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
<System.Web.Script.Services.ScriptService()> _
<WebService(Namespace:="http://tempuri.org/")> _
<WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)> _
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Public Class WebService
Inherits System.Web.Services.WebService
<WebMethod()> _
Public Function GetProducts() As List(Of Product)
Dim products As List(Of Product) = New List(Of Product)()
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim query As String = "SELECT TOP 3 * FROM tblFiles WHERE ContentType = 'image/jpeg'"
Using con As SqlConnection = New SqlConnection(conString)
Dim cmd As SqlCommand = New SqlCommand(query, con)
con.Open()
Dim sdr As SqlDataReader = cmd.ExecuteReader()
While sdr.Read()
products.Add(New Product With {
.Id = Convert.ToInt32(sdr("Id")),
.Name = sdr("Name").ToString(),
.ProdImage = Convert.ToBase64String(CType(sdr("Data"), Byte()), 0, (CType(sdr("Data"), Byte())).Length)
})
End While
con.Close()
End Using
Return products
End Function
Public Class Product
Public Property Id As Integer
Public Property Name As String
Public Property ProdImage As String
End Class
End Class
Screenshot
