Hi mahesh213,
Check this example. Now please take its reference and correct your code.
Database
CREATE TABLE LogDetails
(
LogId INT PRIMARY KEY IDENTITY,
Id INT NOT NULL,
ExceptionMessage VARCHAR(500),
ControllerName VARCHAR(50),
LogTime DATETIME
)
For this example I have used of Northwind database that you can download using the link given below.
I have made use of the following table Customers with the schema as follows.
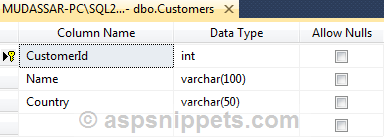
I have already inserted few records in the table.
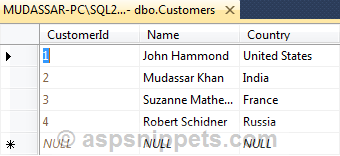
You can download the database table SQL by clicking the download link below.
Download SQL file
Controller
public class HomeController : Controller
{
// GET: /Home/
public ActionResult Index()
{
return View();
}
[HttpPost]
public string AddUser(Customer customer)
{
string status = "";
if (customer != null)
{
try
{
using (TestEntities db = new TestEntities())
{
db.Customers.AddObject(customer);
int returnValue = db.SaveChanges();
if (returnValue > 0)
{
status = "Record added Successfully";
}
}
}
catch (Exception ex)
{
status = "Addition of Record unsucessfull !";
using (TestEntities db = new TestEntities())
{
LogDetail log = new LogDetail();
log.Id = 1;
log.ExceptionMessage = (ex.InnerException).Message;
log.ControllerName = this.ControllerContext.RouteData.Values["controller"].ToString();
log.LogTime = DateTime.Now;
db.LogDetails.AddObject(log);
db.SaveChanges();
}
}
}
return status;
}
}
View
<html>
<head>
<title>Index</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.5.5/angular.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.6.8/angular.min.js"></script>
<script type="text/javascript">
var app = angular.module("myApp", []);
app.controller("myCntrl", ['$scope', '$http', 'myService', function ($scope, $http, myService) {
$scope.AddUpdateUser = function () {
var User = { Name: $scope.Name, Country: $scope.Country };
var getData = myService.AddUser(User);
getData.then(function (msg) {
$scope.msg = msg.data;
if (msg.data == "Record added Successfully") {
ClearFields();
}
else {
$scope.Name = $scope.Name;
$scope.Country = $scope.Country;
}
}, function (msg) {
$scope.msg = msg.data;
});
}
function ClearFields() {
$scope.Name = "";
$scope.Country = "";
}
} ]);
app.service("myService", function ($http) {
this.AddUser = function (user) {
var response = $http({
method: "post",
url: "/Home/AddUser",
params: user,
dataType: "json",
headers: { "Content-Type": "application/json" }
});
return response;
}
});
</script>
</head>
<body ng-app="myApp" ng-controller="myCntrl">
<div class="container">
<div id="wrapper" class="clearfix">
<div class="well">
<form name="userForm" novalidate>
<div class="form-horizontal">
<div class="row">
<div class="col-md-3">
<label for="Name">
Name</label>
<input type="text" class="form-control" ng-model="Name" />
</div>
<div class="col-md-3">
<label for="Country">
Country</label>
<input type="text" class="form-control" ng-model="Country" />
</div>
</div>
<div>
</div>
<div class="form-group" style="width: 120%; text-align: center; padding: 10px;">
<div class="col-md-offset-2 col-md-5">
<p>
<button class="btn btn-success btn-sm" ng-click="AddUpdateUser()">
<span class="glyphicon glyphicon-ok"></span>Submit</button>
</p>
</div>
</div>
<span>Status : {{msg}}</span>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
Screenshots

Inserted record and Log Table
