Hi alya14,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
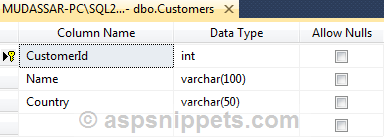
I have already inserted few records in the table.
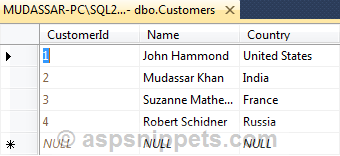
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Namespaces
using System.Configuration;
using System.Data.SqlClient;
Controller
public class HomeController : Controller
{
// GET: /Home/
public ActionResult Index()
{
List<Customer> customers = GetCustomers();
return View(customers);
}
private static List<Customer> GetCustomers()
{
List<Customer> customers = new List<Customer>();
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT * FROM Customers";
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
while (sdr.Read())
{
customers.Add(new Customer
{
Id = Convert.ToInt32(sdr["CustomerId"]),
Name = sdr["Name"].ToString(),
Country = sdr["Country"].ToString()
});
}
con.Close();
}
}
return customers;
}
[HttpPost]
public ActionResult UpdateCustomer(int id, string country)
{
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["constr"].ConnectionString);
con.Open();
SqlCommand cmd = new SqlCommand("SELECT COUNT(*) FROM Customers WHERE CustomerId=@P1", con);
cmd.Parameters.AddWithValue("@P1", id);
int sonuc = Convert.ToInt32(cmd.ExecuteScalar());
con.Close();
if (sonuc >= 1)
{
con.Open();
cmd = new SqlCommand("UPDATE Customers SET Country=@P2 WHERE CustomerId=@P1", con);
cmd.Parameters.AddWithValue("@P1", id);
cmd.Parameters.AddWithValue("@P2", country);
cmd.ExecuteNonQuery();
con.Close();
}
return new EmptyResult();
}
}
View
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<IEnumerable<_ActionLink_Update_Record_MVC.Models.Customer>>" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Index</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$('.Update').on("click", function () {
var id = $(this).closest('tr').find('td').eq(0).html().trim();
var country = $(this).closest('tr').find('#txtCountry').val();
var custromer = {};
custromer.id = id;
custromer.country = country;
$.ajax({
type: "POST",
url: "/Home/UpdateCustomer",
data: custromer,
dataType: "json"
});
return false;
});
});
</script>
</head>
<body>
<table id="myTable" class="table table-striped table-bordered table-condensed">
<thead>
<tr>
<th style="width: 2px">Id</th>
<th>Name</th>
<th>Country</th>
<th></th>
</tr>
</thead>
<tbody>
<% foreach (var customer in Model)
{ %>
<tr>
<td><%: customer.Id%></td>
<td><%: customer.Name%></td>
<td><%:Html.TextBoxFor(t => customer.Country, new { id = "txtCountry", @class = "form-control", style = "width:150px" })%></td>
<td><%:Html.ActionLink("UPDATE", "UpdateManageUser", "Home", new { @class = "Update btn btn-primary pull-left" })%></td>
</tr>
<% } %>
</tbody>
</table>
</body>
</html>
Screenshots

Record in database after update
