Hi RichardSa,
Please refer the sample.
Database
Here i am making use of table tblFiles whose schema is defined as below.
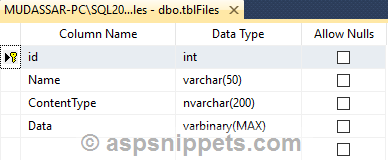
I have inserted few records in the table.

HTML
<asp:GridView ID="gvFiles" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Name" HeaderText="File Name" />
<asp:TemplateField ItemStyle-HorizontalAlign="Center">
<ItemTemplate>
<asp:HiddenField ID="hfId" runat="server" Value='<%#Eval("Id") %>' />
<asp:LinkButton ID="lnkView" Text="View" runat="server" />
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
<hr />
<div id="word-container"></div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript" src="https://unpkg.com/jszip/dist/jszip.min.js"></script>
<script type="text/javascript" src="https://volodymyrbaydalka.github.io/docxjs/dist/docx-preview.js"></script>
<script type="text/javascript">
$(function () {
$('[id*=lnkView]').click(function (e) {
e.preventDefault();
var fileId = $(this).closest('tr').find('[id*=hfId]').val();
$.ajax({
type: "POST",
url: "Default.aspx/GetFile",
data: '{ fileId: "' + fileId + '" }',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
var file = response.d;
PreviewWordDoc(file);
},
failure: function (response) {
alert(response.responseText);
},
error: function (response) {
alert(response.responseText);
}
});
});
});
function Base64ToBytes(base64) {
var s = window.atob(base64);
var bytes = new Uint8Array(s.length);
for (var i = 0; i < s.length; i++) {
bytes[i] = s.charCodeAt(i);
}
return bytes;
};
function PreviewWordDoc(file) {
//Convert Base64 to Byte Array.
var bytes = Base64ToBytes(file.Data);
//Convert Byte Array to File object.
var doc = new File([bytes], file.Name);
//Set the Document options.
var docxOptions = Object.assign(docx.defaultOptions, {
useMathMLPolyfill: true
});
//Reference the Container DIV.
var container = document.querySelector("#word-container");
//Render the Word Document.
docx.renderAsync(doc, container, null, docxOptions);
}
</script>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.Web.Services;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.Web.Services
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandText = "SELECT Id, Name FROM tblFiles WHERE Id IN (9,11)";
cmd.Connection = con;
con.Open();
gvFiles.DataSource = cmd.ExecuteReader();
gvFiles.DataBind();
con.Close();
}
}
}
}
[WebMethod]
public static object GetFile(int fileId)
{
string fileName = string.Empty;
byte[] bytes = null;
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
cmd.Parameters.AddWithValue("@FileID", fileId);
cmd.CommandText = "SELECT Name, Data FROM tblFiles WHERE Id = @FileID";
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
if (sdr.Read())
{
fileName = sdr["Name"].ToString();
bytes = (byte[])sdr["Data"];
}
}
con.Close();
}
}
return new { Name = fileName, Data = Convert.ToBase64String(bytes, 0, bytes.Length) };
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand()
cmd.CommandText = "SELECT Id, Name FROM tblFiles WHERE Id IN (9,11)"
cmd.Connection = con
con.Open()
gvFiles.DataSource = cmd.ExecuteReader()
gvFiles.DataBind()
con.Close()
End Using
End Using
End If
End Sub
<WebMethod>
Public Shared Function GetFile(ByVal fileId As Integer) As Object
Dim fileName As String = String.Empty
Dim bytes As Byte() = Nothing
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand()
cmd.CommandType = CommandType.Text
cmd.Connection = con
cmd.Parameters.AddWithValue("@FileID", fileId)
cmd.CommandText = "SELECT Name, Data FROM tblFiles WHERE Id = @FileID"
con.Open()
Using sdr As SqlDataReader = cmd.ExecuteReader()
If sdr.Read() Then
fileName = sdr("Name").ToString()
bytes = CType(sdr("Data"), Byte())
End If
End Using
con.Close()
End Using
End Using
Return New With {Key _
.Name = fileName, Key _
.Data = Convert.ToBase64String(bytes, 0, bytes.Length)
}
End Function
Screenshot
