Hi MattNorman,
Please refer below code.
Database
I have made use of the following table Customers with the schema as follows.
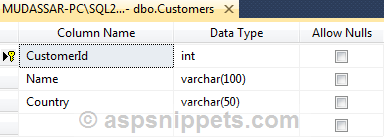
I have already inserted few records in the table.
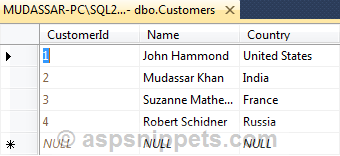
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<div id="simpleModal" class="modal" tabindex="-1" role="dialog">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">
Customer Details Form</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="CustomerId" HeaderText="CustomerId" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
<asp:TemplateField>
<ItemTemplate>
<asp:CheckBox ID="cbCountry" runat="server" />
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</div>
<div class="modal-footer">
<asp:Button ID="btnSubmit" runat="server" Text="Submit" OnClick="Submit" />
<button type="button" class="btn btn-secondary" data-dismiss="modal">
Close</button>
</div>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js"
integrity="sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN"
crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm"
crossorigin="anonymous" />
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"
integrity="sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl"
crossorigin="anonymous"></script>
<script type="text/javascript">
window.onload = function () {
OpenBootstrapPopup();
};
function OpenBootstrapPopup() {
$("#simpleModal").modal('show');
}
</script>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindGrid();
}
}
protected void Submit(object sender, EventArgs e)
{
string message = "Checked Ids are:\\n";
foreach (GridViewRow row in gvCustomers.Rows)
{
CheckBox chk = (row.FindControl("cbCountry") as CheckBox);
if (chk.Checked)
{
message += row.Cells[0].Text + "\\n";
}
}
ClientScript.RegisterStartupScript(this.GetType(), "alert", "alert('" + message + "');", true);
}
private void BindGrid()
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM Customers", con))
{
con.Open();
gvCustomers.DataSource = cmd.ExecuteReader();
gvCustomers.DataBind();
con.Close();
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGrid()
End If
End Sub
Protected Sub Submit(ByVal sender As Object, ByVal e As EventArgs)
Dim message As String = "Checked Ids are:\n"
For Each row As GridViewRow In gvCustomers.Rows
Dim chk As CheckBox = (TryCast(row.FindControl("cbCountry"), CheckBox))
If chk.Checked Then
message += row.Cells(0).Text & "\n"
End If
Next
ClientScript.RegisterStartupScript(Me.GetType(), "alert", "alert('" & message & "');", True)
End Sub
Private Sub BindGrid()
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT * FROM Customers", con)
con.Open()
gvCustomers.DataSource = cmd.ExecuteReader()
gvCustomers.DataBind()
con.Close()
End Using
End Using
End Sub
Screenshot
