Hi vivekmairothi...,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
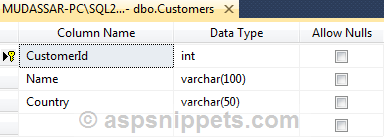
I have already inserted few records in the table.
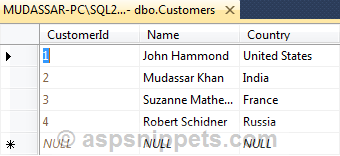
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:ScriptManager runat="server" />
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false" OnRowDataBound="OnRowDataBound">
<Columns>
<asp:BoundField DataField="CustomerId" HeaderText="Id" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
<asp:BoundField DataField="" HeaderText="Action" />
</Columns>
</asp:GridView>
<asp:Panel ID="pnlEdit" runat="server" CssClass="modalPopup" Style="display: none">
<asp:Label runat="server" Text="Customer Details" Font-Bold="true"></asp:Label>
<br />
<br />
<table align="center">
<tr>
<td>
<asp:Label ID="lblId" runat="server" Text="CustomerId"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtCustomerID" runat="server" ReadOnly="true"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label ID="lblName" runat="server" Text="Name"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtName" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label ID="lblCountry" runat="server" Text="Country"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtCountry" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Button ID="btnSave" runat="server" Text="Save" OnClick="OnSave" />
</td>
<td>
<asp:Button ID="btnCancel" runat="server" Text="Cancel" />
</td>
</tr>
</table>
</asp:Panel>
<asp:LinkButton ID="lnkFake" runat="server"></asp:LinkButton>
<cc1:ModalPopupExtender ID="popup" runat="server" DropShadow="false" PopupControlID="pnlEdit"
TargetControlID="lnkFake" BackgroundCssClass="modalBackground">
</cc1:ModalPopupExtender>
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
this.BindGridView();
}
private void BindGridView()
{
string sql = "SELECT CustomerId,Name,Country FROM Customers";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlDataAdapter sda = new SqlDataAdapter(sql, con))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
gvCustomers.DataSource = dt;
gvCustomers.DataBind();
}
}
}
}
protected void OnRowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
LinkButton lnkEdit = new LinkButton();
lnkEdit.ID = "lnkEdit";
lnkEdit.Text = "Edit";
lnkEdit.Click += new EventHandler(OnEdit);
e.Row.Cells[e.Row.Cells.Count - 1].Controls.Add(lnkEdit);
}
}
protected void OnEdit(object sender, EventArgs e)
{
GridViewRow row = (sender as LinkButton).NamingContainer as GridViewRow;
txtCustomerID.Text = row.Cells[0].Text;
txtName.Text = row.Cells[1].Text;
txtCountry.Text = row.Cells[2].Text;
popup.Show();
}
protected void OnSave(object sender, EventArgs e)
{
string customerId = txtCustomerID.Text;
string name = txtName.Text;
string country = txtCountry.Text;
string sql = "UPDATE Customers SET Name=@Name, Country=@Country WHERE CustomerId=@CustomerId";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(sql, con))
{
cmd.Parameters.AddWithValue("@CustomerId", customerId);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
this.BindGridView();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
Me.BindGridView()
End Sub
Private Sub BindGridView()
Dim sql As String = "SELECT CustomerId,Name,Country FROM Customers"
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using sda As SqlDataAdapter = New SqlDataAdapter(sql, con)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
gvCustomers.DataSource = dt
gvCustomers.DataBind()
End Using
End Using
End Using
End Sub
Protected Sub OnRowDataBound(ByVal sender As Object, ByVal e As GridViewRowEventArgs)
If e.Row.RowType = DataControlRowType.DataRow Then
Dim lnkEdit As LinkButton = New LinkButton()
lnkEdit.ID = "lnkEdit"
lnkEdit.Text = "Edit"
AddHandler lnkEdit.Click, AddressOf OnEdit
e.Row.Cells(e.Row.Cells.Count - 1).Controls.Add(lnkEdit)
End If
End Sub
Protected Sub OnEdit(ByVal sender As Object, ByVal e As EventArgs)
Dim row As GridViewRow = TryCast((TryCast(sender, LinkButton)).NamingContainer, GridViewRow)
txtCustomerID.Text = row.Cells(0).Text
txtName.Text = row.Cells(1).Text
txtCountry.Text = row.Cells(2).Text
popup.Show()
End Sub
Protected Sub OnSave(ByVal sender As Object, ByVal e As EventArgs)
Dim customerId As String = txtCustomerID.Text
Dim name As String = txtName.Text
Dim country As String = txtCountry.Text
Dim sql As String = "UPDATE Customers SET Name=@Name, Country=@Country WHERE CustomerId=@CustomerId"
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand(sql, con)
cmd.Parameters.AddWithValue("@CustomerId", customerId)
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Country", country)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
Me.BindGridView()
End Sub
Screenshot
