Hi mahesh213,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
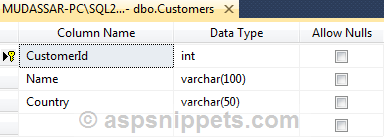
I have already inserted few records in the table.
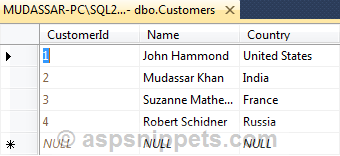
You can download the database table SQL by clicking the download link below.
Download SQL file
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
return View();
}
public JsonResult ValidateUserPassword(string password)
{
CustomerEntities entity = new CustomerEntities();
string[] passParts = Regex.Matches(password, @"[a-zA-Z]+|\d+")
.Cast<Match>()
.Select(m => m.Value)
.ToArray();
string[] users = entity.Customers.Select(x => x.Name).Distinct().ToArray();
bool isValid = true;
for (int i = 0; i < users.Length; i++)
{
string user = users[i];
for (int j = 0; j < passParts.Length; j++)
{
if (user.ToLower().Contains(passParts[j].ToLower()))
{
isValid = false;
return Json(isValid);
}
}
}
return Json(isValid);
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.9/angular.min.js"></script>
<script type="text/javascript">
var app = angular.module("MyApp", []);
app.controller("MyController", function ($scope, $http, $window) {
$scope.Save = function () {
$http({
method: "POST",
url: "/Home/ValidateUserPassword",
params: { password: $scope.txtPassword },
headers: { "Content-Type": "application/json" }
}).then(function (response) {
if (!response.data) {
$window.alert("Password should not contain part of user name.");
}
});
}
});
</script>
</head>
<body ng-app="MyApp" ng-controller="MyController">
<input type="text" ng-model="txtPassword" />
<button type="button" ng-click="Save()">Save</button>
</body>
</html>
Screenshot
