Hi Destinykid,
Check this example. Now please take its reference and correct your code.
Database
I have made use of a table named tblFiles whose schema is defined as follows.
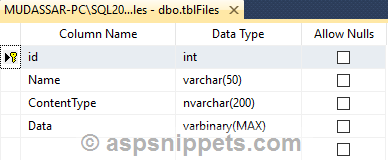
I have already inserted few records in the table.
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Data.SqlClient;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using System.IO;
Model
public class FileModel
{
public int Id { get; set; }
public string Name { get; set; }
public string ContentType { get; set; }
public byte[] Data { get; set; }
}
Controller
public class HomeController : Controller
{
static string constr = @"Data Source=.;Initial Catalog=dbFiles;uid=sa;pwd=pass@123;";
public IActionResult Index()
{
return View(PopulateFiles());
}
[HttpPost]
public IActionResult Index(List<IFormFile> postedFiles)
{
foreach (IFormFile postedFile in postedFiles)
{
string fileName = Path.GetFileName(postedFile.FileName);
string type = postedFile.ContentType;
byte[] bytes = null;
using (MemoryStream ms = new MemoryStream())
{
postedFile.CopyTo(ms);
bytes = ms.ToArray();
}
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandText = "INSERT INTO tblFiles(Name, ContentType, Data) VALUES (@Name, @ContentType, @Data)";
cmd.Parameters.AddWithValue("@Name", fileName);
cmd.Parameters.AddWithValue("@ContentType", type);
cmd.Parameters.AddWithValue("@Data", bytes);
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
return View(PopulateFiles());
}
private static List<FileModel> PopulateFiles()
{
List<FileModel> files = new List<FileModel>();
using (SqlConnection con = new SqlConnection(constr))
{
string query = "SELECT * FROM tblFiles WHERE ContentType = 'image/jpeg' ORDER BY Id DESC";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
files.Add(new FileModel
{
Id = Convert.ToInt32(sdr["Id"]),
Name = sdr["Name"].ToString(),
Data = (byte[])sdr["Data"]
});
}
}
con.Close();
}
}
return files;
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using Upload_Image_Core_MVC.Models
@model List<FileModel>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form asp-action="Index" asp-controller="Home" method="post" enctype="multipart/form-data">
<span>Select File:</span>
<input type="file" name="postedFiles" multiple />
<input type="submit" value="Upload" />
</form>
<hr />
<table cellpadding="0" cellspacing="0">
<tr>
<th>Name</th>
<th>Image</th>
</tr>
@foreach (FileModel file in Model)
{
<tr>
<td>@file.Name</td>
<td>
<img src="data:image/jpeg;base64,@Convert.ToBase64String(file.Data)"
width="100" height="100" />
</td>
</tr>
}
</table>
</body>
</html>
Screenshot
