Hi rani,
You can directly DataTable as model in the view. If you want to pass DataTable with other object then only you need to create a model class with DataTable as property and set the property and pass the model to the view.
Check this example. Now please take its reference and correct your code.
Controller
public class HomeController : Controller
{
public IActionResult Index()
{
DataTable dt = new DataTable();
dt.Columns.AddRange(new DataColumn[3] {
new DataColumn("Id"),
new DataColumn("Name"),
new DataColumn("Country") });
dt.Rows.Add(1, "John Hammond", "United States");
dt.Rows.Add(2, "Mudassar Khan", "India");
dt.Rows.Add(3, "Suzanne Mathews", "France");
dt.Rows.Add(4, "Robert Schidner", "Russia");
return View(dt);
}
}
View
@using System.Data;
@model DataTable
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<table cellpadding="0" cellspacing="0">
<tr>
<th>CustomerId</th>
<th>Name</th>
<th>Country</th>
</tr>
@foreach (DataRow row in Model.Rows)
{
<tr>
<td>@row["Id"]</td>
<td>@row["Name"]</td>
<td>@row["Country"]</td>
</tr>
}
</table>
</body>
</html>
Screenshot
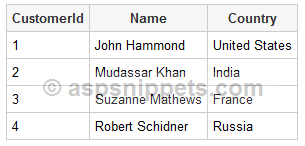