Hi Mohal,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
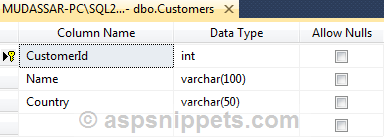
I have already inserted few records in the table.
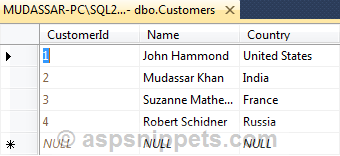
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Code
C#
private void BindGrid()
{
string constring = @"Data Source=192.168.0.100\SQL2019;Initial Catalog=Northwind;User id = sa;password=pass@123";
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("SELECT TOP 5 CustomerId,ContactName,Country FROM Customers", con))
{
cmd.CommandType = CommandType.Text;
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
dataGridView1.DataSource = dt;
}
}
}
}
}
private void Compare(object sender, EventArgs e)
{
DataTable dataTable = new DataTable();
dataTable.Columns.Add("Column0");
dataTable.Columns.Add("Column1");
dataTable.Columns.Add("Column2");
foreach (DataGridViewRow row in dataGridView1.Rows)
{
DataRow dr = dataTable.NewRow();
int column = 0;
for (int j = 0; j < dataGridView1.Columns.Count; j++)
{
if (row.Cells[j].Value != null)
{
dr["Column" + j.ToString()] = row.Cells[j].Value.ToString();
}
else
{
column++;
}
}
if (column != dataGridView1.Columns.Count)
{
dataTable.Rows.Add(dr);
}
}
dataGridView2.DataSource = dataTable;
}
VB.Net
Private Sub BindGrid()
Dim constring As String = "Data Source=192.168.0.100\SQL2019;Initial Catalog=Northwind;User id = sa;password=pass@123"
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand("SELECT TOP 5 CustomerId,ContactName,Country FROM Customers", con)
cmd.CommandType = CommandType.Text
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
dataGridView1.DataSource = dt
End Using
End Using
End Using
End Using
End Sub
Private Sub Compare(ByVal sender As Object, ByVal e As EventArgs) Handles button1.Click
Dim dataTable As DataTable = New DataTable()
dataTable.Columns.Add("Column0")
dataTable.Columns.Add("Column1")
dataTable.Columns.Add("Column2")
For Each row As DataGridViewRow In dataGridView1.Rows
Dim dr As DataRow = dataTable.NewRow()
Dim column As Integer = 0
For j As Integer = 0 To dataGridView1.Columns.Count - 1
If row.Cells(j).Value IsNot Nothing Then
dr("Column" & j.ToString()) = row.Cells(j).Value.ToString()
Else
column += 1
End If
Next
If column <> dataGridView1.Columns.Count Then
dataTable.Rows.Add(dr)
End If
Next
dataGridView2.DataSource = dataTable
End Sub
Screenshot
